Table of Contents
Have you ever wanted to turn your WooCommerce variable products into affiliate items with specific external links for each variation? While you can easily designate a product as an external or affiliate type by selecting “External/Affiliate” product type. But this method only allows for a single URL.
In this post, we address the limitation for variable products that require different URLs for each variation. The code creates a distinct URL field and custom button text field for each product variation in the edit product section, enabling store owners to set unique external links that open in a new tab after the customer chooses the variation and clicks the ‘Add to Cart’ button. By implementing this solution, you can enhance the shopping experience for your customers while maximizing your affiliate potential for variable products as well.
Solution: Add External Links for Each Variations and Open it in New Tab for WooCommerce Variable Products
The code will adds the text URL field for each variations in the edit product page at the backend.
If an url is entered for the variation, the value gets saved. On the product page when customers select a variation and click on ‘Add to cart’, the external link will be opened in new tab.
// Add External URL Field and Custom Button Text Field to Product Variations add_action('woocommerce_variation_options', 'ts_add_fields_to_variations', 10, 3); function ts_add_fields_to_variations($loop, $variation_data, $variation) { // External URL Field woocommerce_wp_text_input(array( 'id' => "external_url_{$variation->ID}", 'name' => "external_url_{$variation->ID}", 'label' => __('External URL', 'woocommerce'), 'placeholder' => 'https://example.com', 'value' => get_post_meta($variation->ID, '_external_url', true), )); // Custom Button Text Field woocommerce_wp_text_input(array( 'id' => "button_text_{$variation->ID}", 'name' => "button_text_{$variation->ID}", 'label' => __('Custom Add to Cart Button Text', 'woocommerce'), 'placeholder' => 'Buy at partnersite.com', 'value' => get_post_meta($variation->ID, '_button_text', true), )); } // Save External URL and Custom Button Text Field Value add_action('woocommerce_save_product_variation', 'ts_save_variation_fields', 10, 2); function ts_save_variation_fields($variation_id, $i) { $external_url = isset($_POST["external_url_{$variation_id}"]) ? $_POST["external_url_{$variation_id}"] : ''; $button_text = isset($_POST["button_text_{$variation_id}"]) ? $_POST["button_text_{$variation_id}"] : ''; update_post_meta($variation_id, '_external_url', esc_url($external_url)); update_post_meta($variation_id, '_button_text', sanitize_text_field($button_text)); } // Pass External URL and Button Text to the Frontend add_filter('woocommerce_available_variation', 'ts_add_fields_to_variation'); function ts_add_fields_to_variation($variation_data) { $external_url = get_post_meta($variation_data['variation_id'], '_external_url', true); $button_text = get_post_meta($variation_data['variation_id'], '_button_text', true); if ($external_url) { $variation_data['external_url'] = $external_url; // Add the external URL to the variation data } if ($button_text) { $variation_data['button_text'] = $button_text; // Add the button text to the variation data } return $variation_data; } // JavaScript to handle "Add to Cart" click for external URL and custom button text add_action('wp_footer', 'ts_add_custom_js'); function ts_add_custom_js() { if (is_product()) { ?> <script type="text/javascript"> jQuery(document).ready(function($) { var form = $('form.variations_form'); var button = $('button.single_add_to_cart_button'); // Function to update button text function updateButton(variation) { if (variation && variation.button_text) { button.text(variation.button_text); } else { button.text('Add to Cart'); // Default text } } // When a variation is found form.on('found_variation', function(event, variation) { updateButton(variation); }); // When variations are reset form.on('reset_data', function() { button.text('Add to Cart'); // Reset to default }); // On page load, check if a variation is selected var current_variation = form.find('input[name="variation_id"]').val(); if (current_variation) { var variationData = form.data('product_variations'); if (variationData) { var variation = variationData.find(function(v) { return v.variation_id == current_variation; }); updateButton(variation); } } // When the Add to Cart button is clicked form.on('submit', function(event) { var variation_id = form.find('input[name="variation_id"]').val(); var variation = form.data('product_variations') ? form.data('product_variations').find(function(v) { return v.variation_id == variation_id; }) : null; // Check if the external URL exists if (variation && variation.external_url) { event.preventDefault(); // Prevent the default form submission // Open the external URL in a new tab window.open(variation.external_url, '_blank'); } // Else, submit the form normally }); }); </script> <?php } }
Output
The code will add a new text fields on the admin edit product page which allows store owners to set an external url and custom button text for product variations as shown in the image below. As shown below, you can add any affiliate link URL and customize the button text accordingly.
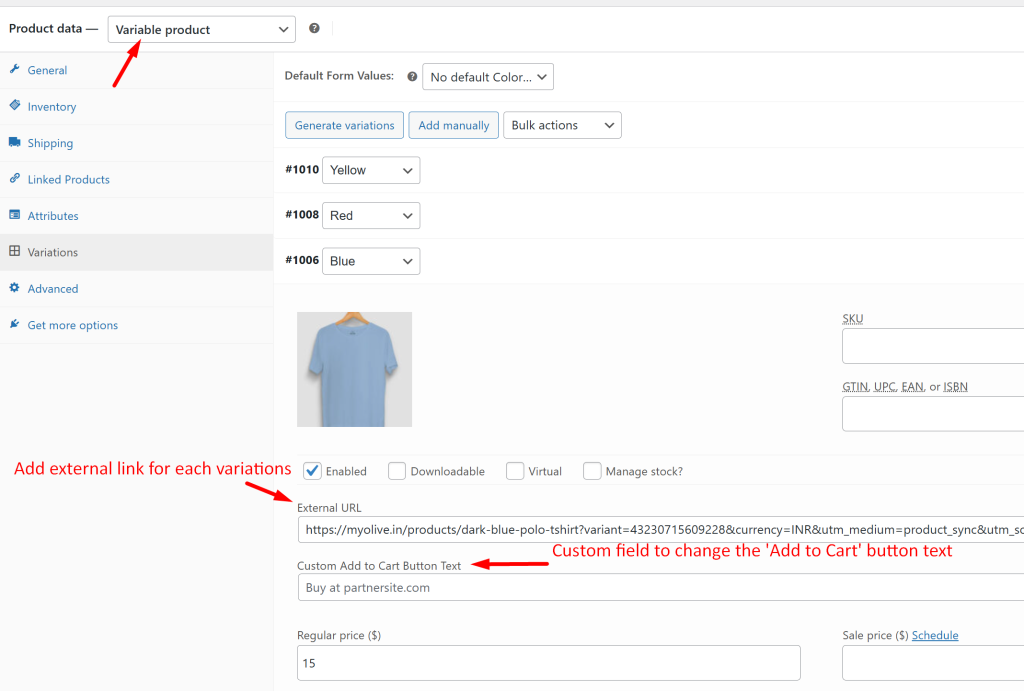
Display Custom Button Text Value on the Product Page
When the customer selects the blue variation on the frontend, the custom button text entered in the button text field will be displayed.
When customers clicks on the ‘Add to cart’ button associated with the variable product, they will be redirected to the specified external link in a new tab instead of being directed to the cart page of your site.
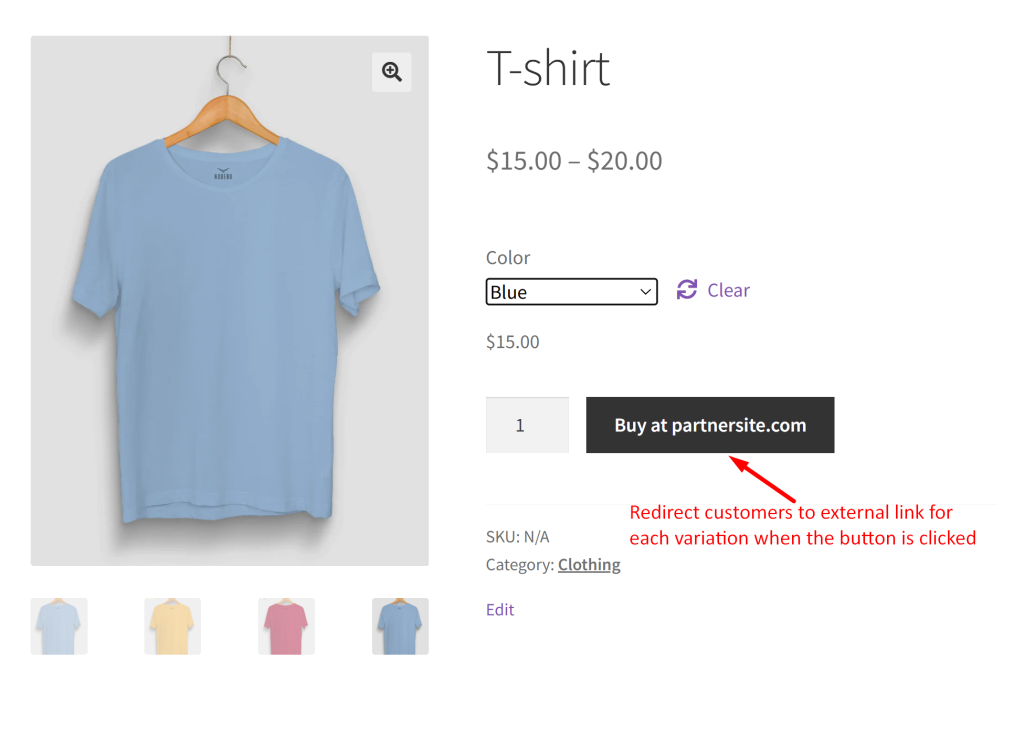
Solution: Add External Links for Each Variations and Open it in Same Tab
In the original code, the external URL is set to open in a new tab by default using the window.open
method. However, if you’d like to change this behavior and make the external URL open in the same tab instead of a new one, you can modify the JavaScript code. Locate this specific line of code from the javascript code:
// Open the external URL in a new tab window.open(variation.external_url, '_blank');
Change the above line of code to this one:
// Open the external URL in same tab window.location.href= variation.external_url;
This updated line of code will redirect the current tab to the external URL, effectively opening the external links of variations in the same window.
Integrate External Links with Bulk Editing Plugins
The above code snippet adds custom fields for external URLs and custom button texts directly in the WooCommerce product variation settings. But imagine a store owner having 300+ variations, and in that case, it is a daunting task to edit the custom fields of each variation. So to optimize this workflow, let’s explore how to make it compatible with bulk editing plugins such as BEAR – WooCommerce Bulk Editor and Products Manager Professional.
Follow the steps below to add and save custom meta key values (_external_url and _button_text) using the plugin:
- When using this plugin, it is crucial that the custom meta key values (_external_url and _button_text) are added and saved in the Meta Fields tab as shown below.
- Make sure these fields are enabled in the Settings tab.
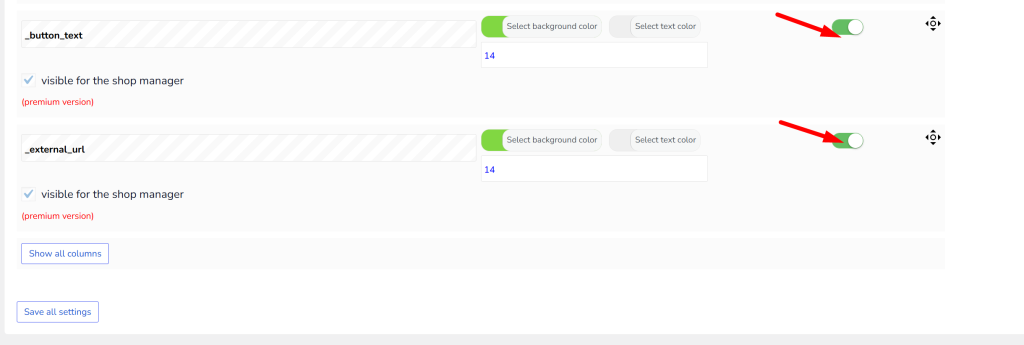
- Return to the product editor and you should now see additional columns for the custom meta fields you enabled.
- Use the bulk selection option to choose multiple products at once.
- Enable variations and bind editing options as needed.
- In the newly visible columns for _external_url and _button_text, directly enter or modify the values for any one selected product variation and press enter.
- The plugin will add the values in bulk and save the updated values for all the selected products, ensuring that the custom meta key values are correctly stored in the WooCommerce database.
- Check the modified custom field values on the frontend of the variable product page to ensure they display correctly.
We hope you’ve successfully added unique external links for each variation of your products, as this paves the way to increase your affiliate potential. Additionally, consider applying this strategy to make WooCommerce external products, images, and titles open in a new tab . By doing so, you create a smoother browsing experience for your customers, which can lead to increased satisfaction and retention. Plus, minimizing bounce rates can positively impact your site’s SEO, helping you attract more visitors over time.
Hi Great bit of code.
I was a bit confused that on my site it actually added the items to the cart on my site as well as redirected me to the link, is that supposed to happen or have I misunderstood something?
Hi Shane,
I tried to replicate the issue on my end, but the code doesn’t add the item to the WooCommerce cart. You can refer to this video link: https://go.screenpal.com/watch/cZXIfRnVhlZ.
Try disabling all other plugins except WooCommerce and temporarily switching to a default theme to see if any of them might be affecting this behavior. If the issue’s still popping up, could you let us know about any other customizations you’ve made for variations? That’ll help us sort it out more easily!
Thank you so much for this. Much needed and appreciated. Is it possible to add a field for an optional button text for each variation? i.e. buy “buy at partnersite.com”
Also compatibility with WPC Variation Bulk Editor for WooCommerce? This would allow setting many many links at once and save a ton of time.
Hi paul,
Glad that it works well for you! I have updated the post to add optional button text and please check the revised code. Also, I have checked the code with the plugin that you have mentioned but the custom field values added in the plugin’s interface are not getting saved in the database. For detailed assistance in making the code snippet compatible with the WPC Variation Bulk Editor, I recommend reaching out to the plugin support team.
Thank you, Saranya! You are amazing!!
I will reach out to WPC Variation Bulk Editor. Do you know of any other bulk editing plugin that might be compatible with the external URL field for the variations? Some of my products have 300 variations.
Found a plugin that will allow me to bulk edit the variations’ external URL and button text fields using the _external_url and _button_text from your snippet 🙂
Plugin: Admin Columns – https://www.admincolumns.com/
Screenshot: https://share.zight.com/geuKK9g4
Hi Paul,
Thank you for your reply! Glad to know that you found a plugin that works with the _external_url and _button_text fields from the snippet.
Unfortunately trying bulk edit too many variations at once using the Admin Columns plugin results in the custom fields being populated with URL and button text in the product backend and the button text showing on the front end but the products still end up in the cart when clicking 🙁
Now the fields are populated but it doesn’t work. Scratching my head. Definitely a database issue.
Hi Paul,
We have found a compatible plugin that works with the code, allowing bulk editing of custom fields, specifically for the _external_url and _button_text custom field values. We have updated our post and you can find the detailed steps on how to implement this functionality with a plugin below the topic titled as “Integrate External Links with Bulk Editing Plugins”.
Hi Saranya,
Thank you again. You are amazing!
I am worried the problem is more with my database or theme. When there are too many variations in a product the external link is not triggered and the variation is added to the cart regardless of how I added the link to the custom field (bulk editor or normal entry). I am not sure what the threshold is for the # of variations is for when it stops working.
Sample URL where it doesn’t work: https://deathwaiver.com/product/ten-thousand-interval-short/
Hi Saranya, Thank you again for your amazing help! I was having problems with products that had many variations and the external URL would not work. The product was still added to the cart. I don’t think this was related to bulk editor as the URL was present regardless of how it was added (bulk or individually). Chat GPT modified the code somewhat and now it seems to work consistently!! Your code may need to be modified somewhat to address products with many variations. Maybe a site’s server or hosting specs has something to do with it? Anyway here is… Read more »