In WordPress, when you create new content, such as blog posts, product pages, and images, they’re categorized as post types. These post types receive sequential IDs in the wp_posts table of the database. Where things get disruptive in this default sequential order numbering system is when post types are inserted or uploaded between new WooCommerce orders.
This issue often causes WooCommerce order numbers to break, and that’s why you will see non-sequential orders in your WooCommerce orders table. To effectively address this, we’ve meticulously outlined a proven, straightforward WooCommerce customization solution in this post.
Solution: Add Sequential Custom Order Numbers in WooCommerce
The code snippet will add sequential order numbers to the WooCommerce Order IDs. The code also provides you the flexibility to specify the desired number of digits (e.g. $digits = 4;) for the order number. For example, if the current sequential order number is 1, the order number will be padded with zeros to make it 0001
.
add_filter( 'woocommerce_checkout_create_order', 'ts_save_order_number_metadata' ); function ts_save_order_number_metadata( $order ) { $digits = 4; // Number of desired digits for the number part $data = get_option('wc_sequential_order_number'); // Get order number sequential helper registered data $number = isset($data['sequential']) ? intval($data['sequential']) + 1 : 1; $data['sequential'] = $number; // Update order number sequential helper registered data update_option('wc_sequential_order_number', $data); // Add order number as custom metadata $order->add_meta_data('_order_number', str_pad($number, $digits, '0', STR_PAD_LEFT), true); } // Read the order number from metadata add_filter( 'woocommerce_order_number', 'ts_define_order_number', 10, 2 ); function ts_define_order_number( $order_id, $order ) { if ( $order_number = $order->get_meta('_order_number') ) { $order_id = $order_number; } return $order_id; }
Output
When a new order is created during the checkout process, the code retrieves the current sequential order number from the database (stored in the wc_sequential_order_number option), increments it by 1, and then saves it back to the database. It also adds the order number as custom metadata to the order object, padding it with zeros to achieve the desired number of digits.
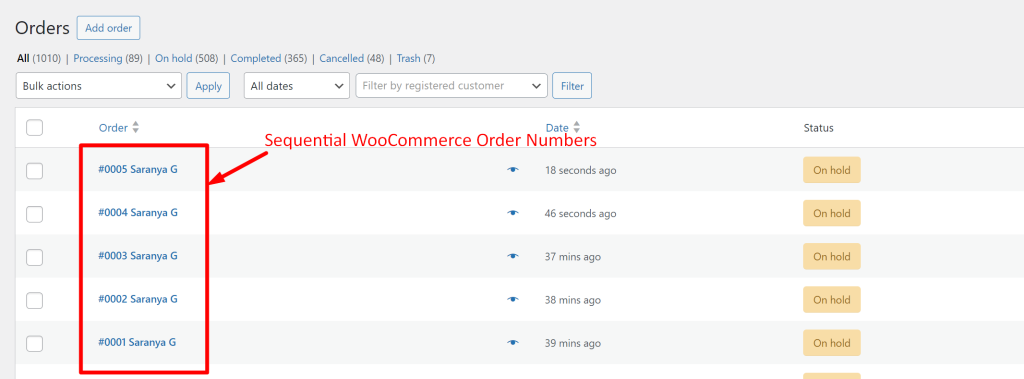
If you want to add some extra flair to your WooCommerce order numbers, like adding a special prefix or suffix, then it’s just a few steps away. Check out here to add prefix or suffix to WooCommerce orders.