Table of Contents
Some products may be larger than standard size and for such products, you can offer special shipping arrangements when they go beyond a particular size. Foe example, products like furniture, large canvases, or bulky equipment can sometimes be too big for standard shipping options. In these cases, store owners need to adjust the shipping methods to make sure they match the size of the product. By limiting shipping options, customers are guided to choose the right method for their purchase.
In this guide, we’ll show you how to enable or disable shipping methods based on the size of a single product or the total size of everything in the cart.
Enable or Disable Shipping Based on Individual Product Dimensions in WooCommerce
This code will disable the standard WooCommerce shipping methods for such products that have dimensions greater than the specified size.
add_filter('woocommerce_package_rates', 'ts_custom_shipping_methods', 10, 2); function ts_custom_shipping_methods($rates, $package) { // Check if any product in the cart has dimensions greater than 40*40*40 $has_large_product = false; foreach ($package['contents'] as $cart_item) { $product = wc_get_product($cart_item['product_id']); if ($product && $product->is_visible() && $product->get_length() > 40 && $product->get_width() > 40 && $product->get_height() > 40) { $has_large_product = true; break; } } // Adjust shipping methods based on the presence of large products if ($has_large_product) { // If large products are present, hide all shipping methods except free shipping $rates = ts_hide_shipping_when_free_is_available($rates); } return $rates; } // Function to hide shipping methods when free shipping is available function ts_hide_shipping_when_free_is_available($rates) { $free = array(); foreach ($rates as $rate_id => $rate) { if ('free_shipping' === $rate->get_method_id()) { $free[$rate_id] = $rate; } } return !empty($free) ? $free : $rates; }
Output
Let’s see how the dimension of a product is set from the admin side with the following steps mentioned below.
- From the WordPress admin sidebar, click on “Products”.
- Locate the product you want to set the dimensions and click on its name or “Edit” link.
- Under “Product Data” select “Shipping”, find fields for dimensions (length, width, height), enter the appropriate values, and click on Update.
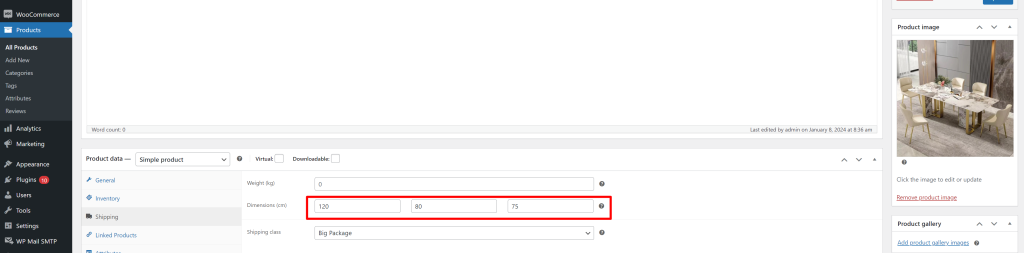
When the cart contains items that are larger than the maximum dimensions specified in the code, then the code implies hiding all shipping methods except ‘free shipping’.
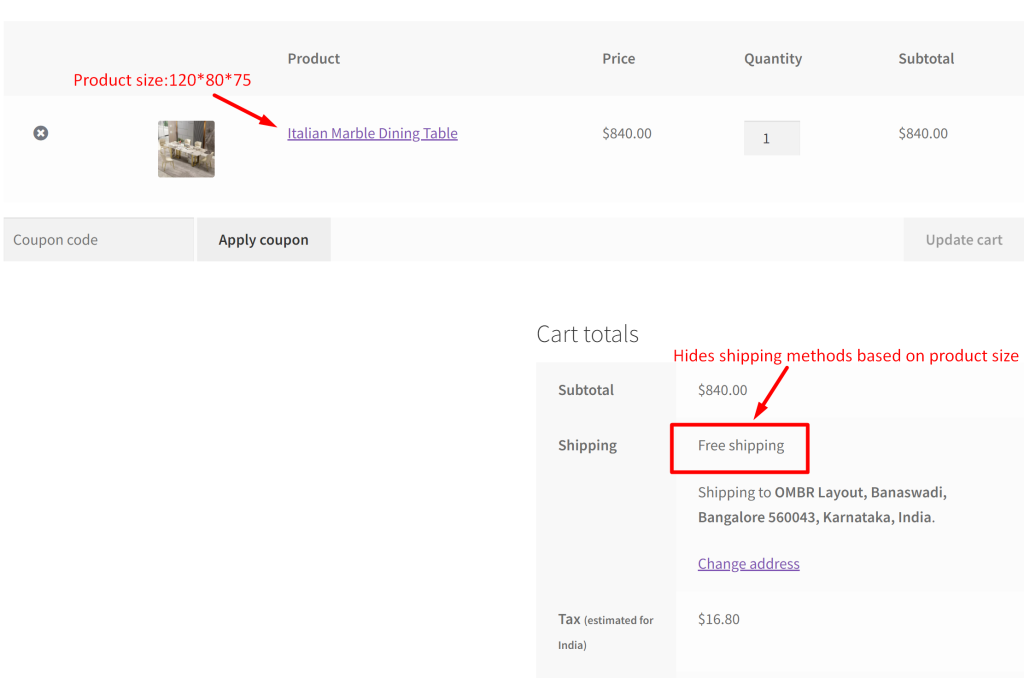
When the cart contains items with a product size smaller than the specified dimensions, all available shipping methods are displayed.
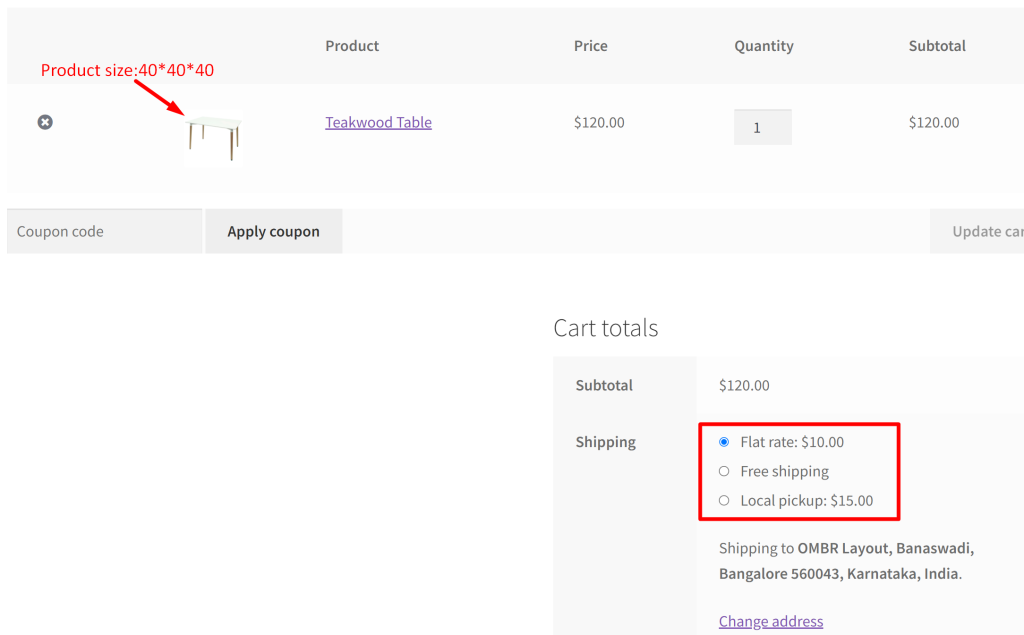
Enable or Disable Shipping Based on Total Product Dimensions in WooCommerce Cart
Products like furniture, large canvases, or bulky equipment often exceed standard shipping size limits. In such cases, store owners need to consider the practical difficulties and adjust shipping methods accordingly.Limiting shipping options ensures customers select methods that match the product’s requirements.In this guide, we’ll show how to enable or disable shipping methods based on either the size of a single product or the combined size of everything in the cart.
add_filter('woocommerce_package_rates', 'ts_custom_shipping_methods', 10, 2); function ts_custom_shipping_methods($rates, $package) { // Initialize variables to sum the dimensions of all products in the cart $total_length = 0; $total_width = 0; $total_height = 0; // Loop through each product in the cart to get dimensions foreach ($package['contents'] as $cart_item) { $product = wc_get_product($cart_item['product_id']); if ($product && $product->is_visible()) { // Sum up the dimensions of all items in the cart $total_length += $product->get_length(); $total_width += $product->get_width(); $total_height += $product->get_height(); } } // Check if the total dimensions exceed the specified limits if ($total_length > 100 || $total_width > 100 || $total_height > 100) { // If total dimensions exceed the threshold, hide all shipping methods except free shipping $rates = ts_hide_shipping_when_free_is_available($rates); } return $rates; } // Function to hide shipping methods when free shipping is available function ts_hide_shipping_when_free_is_available($rates) { $free = array(); foreach ($rates as $rate_id => $rate) { if ('free_shipping' === $rate->get_method_id()) { $free[$rate_id] = $rate; } } return !empty($free) ? $free : $rates; }
Output
Let’s create hypothetical dimensions for the two products as an example:
1. Tree Painting Canvas
- Dimensions:
- Length: 60 cm
- Width: 40 cm
- Height: 2 cm
2. White Buddha Canvas
- Dimensions:
- Length: 70 cm
- Width: 50 cm
- Height: 2 cm
With these dimensions, if both canvases are added to the cart, the combined dimensions (if totaling each measurement individually) would be:
- Total Length: 130 cm
- Total Width: 90 cm
- Total Height: 4 cm
Let’s see the condition when two smaller dimension items are added to the cart. Two Smaller Items in the Cart (e.g., White Buddha Canvas and Simple Wall Art)
White Buddha Canvas
- Dimensions: Length: 70 cm, Width: 50 cm, Height: 2 cm
- Simple Wall Art
- Dimensions: Length: 20 cm, Width: 20 cm, Height: 5 cm
When these two items are in the cart:
- Total Length: 70 cm + 20 cm = 90 cm
- Total Width: 50 cm + 20 cm = 70 cm
- Total Height: 2 cm + 5 cm = 7 cm
Since the combined dimensions of both products in cart do not exceed the specified limit of 100 cm, all shipping options will be available for this use case.
This combined total may trigger a restriction based on the overall dimensions, limiting the available shipping methods, particularly if any dimension exceeds the 100 cm limit. In this code, the ‘Free Shipping’ and ‘Local Pickup’ options are disabled when the total dimensions exceed 100 cm in any direction.
Similar to the above functionality, we can also hide shipping methods based on product weight. You can refer to our guide that will hide WooCommerce shipping methods for certain conditions such as weight, order total, product quantity, etc.
This snippet can sum the dimensions for all products on cart?
Hi Bill,
The present code snippet does not sum the dimensions for all products in the cart as it works based on individual product dimensions. We’ve updated the post and you can find the code below the title ‘Enable or Disable Shipping Based on Total Product Dimensions in WooCommerce Cart’ to better address your needs. Let us know if you have any more questions or feedback!